Chapter 3
MATLAB 5.0 Enhancements
-
MATLAB 5.0 Enhancements
-
New Data Constructs
-
New and Enhanced Language Functions
-
New Data Analysis Features
-
New and Enhanced Handle Graphics Features
-
New and Enhanced Handle Graphics Object Properties
-
Improvements to Graphical User Interfaces (GUIs)
-
Enhanced Application Program Interface (API)
-
New Platform-Specific Features
MATLAB 5.0 Enhancements
MATLAB 5.0 featured five major areas of new functionality:
Enhanced Programming and Application
Development Tools
MATLAB 5.0 provided new M-file programming enhancements and application development tools that make it easier than ever to develop and maintain applications in MATLAB. Highlights include:
New Data Types, Structures, and Language
Features
MATLAB 5.0 introduced new data types and language improvements. These new features make it easy to build much larger and more complex MATLAB applications.
Faster, Better Graphics and Visualization
MATLAB 5.0 added powerful new visualization techniques and significantly faster graphics using the Z-buffer algorithm. Presentation graphics were also improved to give you more options and control over how you present your data.
More Mathematical and Data Analysis Tools
With more than 500 mathematical, statistical, and engineering functions, MATLAB gives you immediate access to the numeric computing tools you need. New features with MATLAB 5.0 included:
Enhancements to Simulink and Application Toolboxes
Significant upgrades introduced with MATLAB 5.0 are listed below (note that all these products have been upgraded again with MATLAB 5.2):
New Data Constructs
MATLAB 5.0 supports these new data constructs:
In addition, MATLAB 5.0 features character arrays that incorporate an improved storage method for string data.
Multidimensional Arrays
Arrays (other than sparse matrices) are no longer restricted to two dimensions. You can create and access arrays with two or more dimensions by:
MATLAB functions like zeros
, ones
, and rand
have been extended to accept more than two dimensions as arguments. To create a 3-by-4-by-5 array of ones, for example, use
A = ones(3,4,5)
The new cat
function enables you to concatenate arrays along a specified dimension. For example, create two rectangular arrays A
and B
:
A = [1 2 3; 4 5 6];
B = [6 2 0; 9 1 3];
To concatenate these along the third dimension:
C = cat(3,A,B)
C(:,:,1) =
1 2 3
4 5 6
C(:,:,2) =
6 2 0
9 1 3
You can also create an array with two or more dimensions in which every element has the same value using the repmat
function. repmat
accepts the value with which to fill the array, followed by a vector of dimensions for the array. For example, to create a 2-by-2-by-3-by-3 array B
where every element has the value pi
:
B = repmat(pi,[2 2 3 3]);
You can also use repmat
to replicate or "tile" arrays in a specified configuration.
Function
|
Description
|
cat
|
Concatenate arrays.
|
flipdim
|
Flip array along specified dimension.
|
ndgrid
|
Generate arrays for multidimensional functions and interpolation.
|
ndims
|
Number of array dimensions.
|
permute, ipermute
|
Permute the dimensions of a multidimensional array.
|
reshape
|
Change size.
|
shiftdim
|
Shift dimensions.
|
squeeze
|
Remove singleton array dimensions.
|
sub2ind,ind2sub
|
Single index from subscripts; subscripts from linear index.
|
Cell Arrays
Cell arrays have elements that are containers for any type of MATLAB data, including other cells. You can build cell arrays using:
Structures
Structures are constructs that have named fields containing any kind of data. For example, one field might contain a text string representing a name (patient.name = 'Jane Doe')
, another might contain a scalar representing a billing amount (patient.billing = 127.00)
, and a third might hold a matrix of medical test results. You can organize these structures into arrays of data. Create structure arrays by using individual assignment statements or the new struct
function.
Function
|
Description
|
fieldnames
|
Field names of structure array.
|
getfield
|
Get field of structure array.
|
rmfield
|
Remove structure fields.
|
setfield
|
Set field of structure array.
|
struct
|
Create structure array.
|
struct2cell
|
Structure to cell array conversion.
|
MATLAB Objects
Object-oriented programming within the MATLAB environment was introduced with MATLAB 5.0.
Objects
The MATLAB programming language does not require the use of data types. For many applications, however, it is helpful to associate specific attributes with certain categories of data. To facilitate this, MATLAB allows you to work with objects. Objects are typed structures. A single class name identifies both the type of the structure and the name of the function that creates objects belonging to that class.
Objects differ from ordinary structures in two important ways:
The structure fields of objects are not visible from the command line. Instead, you can access structure fields only from within a method, an M-file associated with the object class. Methods reside in class directories. Class directories have the same name as the class, but with a prepended @
symbol. For example, a class directory named @inline
might contain methods for a class called inline
.
You can create methods that override existing M-files. If an object calls a function, MATLAB first checks to see if there is a method of that name before calling a supplied M-file of that name. You can also provide methods that are called for MATLAB operators. For objects a
and b
, for instance, the expression a + b
calls the method plus(a,b)
if it exists.
Function
|
Description
|
class
|
Create or return class of object.
|
isa
|
True if object is a given class.
|
inferiorto
|
Inferior class relationship.
|
superiorto
|
Superior class relationship.
|
Character Arrays
Strings take up less memory than they did previously: MATLAB 4 required 64 bits per character for string data, whereas MATLAB 5.0 (and later releases) requires only 16 bits per character.
Function
|
Description
|
base2dec
|
Convert base B to decimal number.
|
bin2dec
|
Convert binary to decimal number.
|
char
|
Convert numeric values to string.
|
dec2base
|
Convert decimal number to base.
|
dec2bin
|
Convert decimal to binary number.
|
mat2str
|
Convert a matrix into a string.
|
strcat
|
String concatenation.
|
strmatch
|
Find possible matches for a string.
|
strncmp
|
Compare the first n characters of two strings.
|
strvcat
|
Vertical concatenation of strings.
|
Programming Capabilities
MATLAB 5.0 includes flow-control improvements and new M-file programming tools.
Flow-Control Improvements
MATLAB 5.0 features:
The switch
statement is a convenient way to execute code conditionally when you have many possible cases to choose from. It is no longer necessary to use a series of elseif
statements:
switch input_num
case -1
disp('negative one');
case 0
disp('zero');
case 1
disp('positive one');
otherwise
disp('other value');
end
Only the first matching case
is executed.
switch
can handle multiple conditions in a single case
statement by enclosing the case
expression in a cell array. For example, assume method
exists as a string variable:
switch lower(method)
case {'linear','bilinear'}, disp('Method is linear')
case 'cubic', disp('Method is cubic')
case 'nearest', disp('Method is nearest')
otherwise, disp('Unknown method.')
end
Command
|
Description
|
case
|
Case switch.
|
otherwise
|
Default part of switch statement.
|
switch
|
Conditionally execute code, switching among several cases.
|
MATLAB 5.0 evaluates if
expressions more efficiently than MATLAB 4. For example, consider the expression if
a|b
. If a
is true, then MATLAB will not evaluate b
. Similarly, MATLAB won't execute statements following the expression if
a&b
in the event a
is found to be false.
Operator
|
Description
|
iscell
|
True for a cell array.
|
isequal
|
True if arrays are equal.
|
isfinite
|
True for finite elements.
|
islogical
|
True for logical arrays.
|
isnumeric
|
True if input is a numeric array.
|
isstruct
|
True for a structure.
|
logical
|
Convert numeric values to logical vectors.
|
M-File Programming Tools
MATLAB 5.0 added three features to enhance MATLAB's M-file programming capabilities.
Variable Number of Input and Output Arguments
The varargin
and varargout
commands simplify the task of passing data into and out of M-file functions. For instance, the statement function varargout = myfun(A,B)
allows M-file myfun
to return an arbitrary number of output arguments, while the statement function [C,D] = myfun(varargin)
allows it to accept an arbitrary number of input arguments.
Multiple Functions Within an M-File
MATLAB 5.0 supports having subfunctions within the body of an M-file. These are functions that the primary function in the file can access but that are otherwise invisible.
M-File Profiler
This utility lets you debug and optimize M-files by tracking cumulative execution time for each line of code. Whenever the specified M-file executes, the profiler counts how many time intervals each line uses.
Pseudocode M-Files
The pcode
command saves a pseudocode version of a function or script to disk for later sessions. This pseudocode version is ready-to-use code that MATLAB can access whenever you invoke the function.
Function
|
Description
|
addpath
|
Append directory to MATLAB's search path.
|
assignin
|
Assign variable in workspace.
|
edit
|
Edit an M-file.
|
editpath
|
Modify current search path.
|
evalin
|
Evaluate variable in workspace.
|
fullfile
|
Build full filename from parts.
|
inmem
|
Functions in memory.
|
inputname
|
Input argument name.
|
mfilename
|
Name of the currently running M-file.
|
mexext
|
Return the MEX filename extension.
|
pcode
|
Create pseudocode file (P-file).
|
profile
|
Measure and display M-file execution profiles.
|
rmpath
|
Remove directories from MATLAB's search path.
|
varargin, varargout
|
Pass or return variable numbers of arguments.
|
warning
|
Display warning message.
|
web
|
Point Web browser at file or Web site.
|
New and Enhanced Language Functions
MATLAB 5.0 added a large number of new language functions as well as enhancements to existing functions.
Table 3-1: New Elementary and Specialized Math Functions
Function
|
Description
|
airy
|
Airy functions.
|
besselh
|
Bessel functions of the third kind (Hankel).
|
condeig
|
Condition number with respect to eigenvalues.
|
condest
|
1-norm matrix condition estimate.
|
dblquad
|
Numerical double integration
|
mod
|
Modulus (signed remainder after division).
|
normest
|
2-norm estimate.
|
Table 3-2: New Time and Date Functions
Function
|
Description
|
calendar
|
Calendar.
|
datenum
|
Serial date number.
|
datestr
|
Create date string.
|
datetick
|
Date formatted tick labels.
|
datevec
|
Date components.
|
eomday
|
End of month.
|
now
|
Current date and time.
|
weekday
|
Day of the week.
|
Table 3-3: New Ordinary Differential Equation Functions
Function
|
Description
|
ode45, ode23, ode113, ode23s, ode15s
|
Solve differential equations, low- and high-order methods.
|
odefile
|
Define a differential equation problem for ODE solvers.
|
odeget
|
Extract options from an argument created with odeset .
|
odeset
|
Create and edit input arguments for ODE solvers.
|
Table 3-4: New Matrix Functions
Function
|
Description
|
cholinc
|
Incomplete Cholesky factorization.
|
gallery
|
More than 50 new test matrices.
|
luinc
|
Incomplete LU factorization.
|
repmat
|
Replicate and tile an array.
|
sprand
|
Random uniformly distributed sparse matrices.
|
Table 3-5: New Methods for Sparse Matrices
Method
|
Description
|
bicg
|
BiConjugate Gradients method.
|
bicgstab
|
BiConjugate Gradients Stabilized method.
|
cgs
|
Conjugate Gradients Squared method.
|
eigs
|
Find a few eigenvalues and eigenvectors.
|
gmres
|
Generalized Minimum Residual method.
|
pcg
|
Preconditioned Conjugate Gradients method.
|
qmr
|
Quasi-Minimal Residual method.
|
svds
|
A few singular values.
|
Subscripting and Assignment Enhancements
In MATLAB 5.0, you can:
A statement like A(ones([m,n]))
always returns an m
-by-n
array in which each element is A(1)
. In previous versions, the statement returned different results depending on whether A
was or was not an m
-by-n
matrix.
In previous releases, expressions like A(2:3,4:5) = 5
resulted in an error. MATLAB 5.0 automatically "expands" the 5
to be the right size (that is, 5
*ones(2,2)
).
Integer Bit Manipulation Functions
The ops
directory contains commands that permit bit-level operations on integers. Operations include setting and unsetting, complementing, shifting, and logical AND
, OR
, and XOR
.
Dimension Specification for Data Analysis Functions
MATLAB's basic data analysis functions enable you to supply a second input argument. This argument specifies the dimension along which the function operates. For example, create an array A
:
A = [3 2 4; 1 0 5; 8 2 6];
To sum along the first dimension of A
, incrementing the row index, specify 1 for the dimension of operation:
sum(A,1)
ans =
12 4 15
To sum along the second dimension, incrementing the column index, specify 2 for the dimension:
sum(A,2)
ans =
9
6
16
Other functions that accept the dimension specifier
include prod
, cumprod
, and cumsum
.
Wildcards in Utility Commands
The asterisk (*
) can be used as a wildcard in the clear
and whos
commands. This allows you, for example, to clear only variables beginning with a given character or characters, as in
clear A*
Empty Arrays
Earlier versions of MATLAB allowed for only one empty matrix, the 0-by-0 matrix denoted by []
. MATLAB 5.0 provides for matrices and arrays in which some, but not all, of the dimensions are zero. For example, 1
-by-0
, 10
-by-0
-by-20
, and [3 4 0 5 2]
are all possible array sizes.
The two-character sequence []
continues to denote the 0-by-0 matrix. Empty arrays of other sizes can be created with the functions zeros
, ones
, rand
, or eye
. To create a 0-by-5 matrix, for example, use
E = zeros(0,5)
The basic model for empty matrices is that any operation that is defined for m
-by-n
matrices, and that produces a result with a dimension that is some function of m
and n
, should still be allowed when m
or n
is zero. The size of the result should be that same function, evaluated at zero.
For example, horizontal concatenation
C = [A B]
requires that A
and B
have the same number of rows. So if A
is m
-by-n
and B
is m
-by-p
, then C
is m
-by-(n
+p
). This is still true if m
or n
or p
is zero.
Many operations in MATLAB produce row vectors or column vectors. With MATLAB 5.0 it is possible for the result to be the empty row vector
r = zeros(1,0)
or the empty column vector
c = zeros(0,1)
Some MATLAB functions, like sum
and max
, are reductions. For matrix arguments, these functions produce vector results; for vector arguments they produce scalar results. Backwards compatibility issues arise for the argument []
, which in MATLAB 4 played the role of both the empty matrix and the empty vector. In MATLAB 5.0, empty inputs with these functions produce these results:
New Data Analysis Features
MATLAB 5.0 provides an expanded set of basic data analysis functions.
Function
|
Description
|
convhull
|
Convex hull.
|
cumtrapz
|
Cumulative trapezoidal numerical integration.
|
delaunay
|
Delaunay triangularization.
|
dsearch
|
Search for nearest point.
|
factor
|
Prime factors.
|
inpolygon
|
Detect points inside a polygonal region.
|
isprime
|
True for prime numbers.
|
nchoosek
|
All possible combinations of n elements taken k at a time.
|
perms
|
All possible permutations.
|
polyarea
|
Area of polygon.
|
primes
|
Generate a list of prime numbers.
|
sortrows
|
Sort rows in ascending order.
|
tsearch
|
Search for enclosing Delaunay triangle.
|
voronoi
|
Voronoi diagram.
|
MATLAB 5.0 also offers expanded data analysis in the areas of:
Higher-Dimension Interpolation
The new functions interp3
and interpn
let you perform three-dimensional and multidimensional interpolation. ndgrid
provides arrays that can be used in multidimensional interpolation.
Function
|
Description
|
interp3
|
Three-dimensional data interpolation (table lookup).
|
interpn
|
Multidimensional data interpolation (table lookup).
|
ndgrid
|
Generate arrays for multidimensional functions and interpolation.
|
griddata Based on Delaunay Triangulation
griddata
supports triangle-based interpolation using nearest neighbor, linear, and cubic techniques. It creates smoother contours on scattered data using the cubic interpolation method.
Set Theoretic Functions
The functions union
, intersect
, ismember
, setdiff
, and unique
treat vectors as sets, allowing you to perform operations like union
, intersection
, and difference
of such sets. Other set-theoretical operations include location of common set elements (ismember
) and elimination of duplicate elements (unique
).
Function
|
Description
|
intersect
|
Set intersection of two vectors.
|
ismember
|
Detect members of a set.
|
setdiff
|
Return the set difference of two vectors.
|
setxor
|
Set XOR of two vectors.
|
union
|
Set union of two vectors.
|
unique
|
Unique elements of a vector.
|
New and Enhanced Handle Graphics Features
MATLAB 5.0 features significant improvements to Handle Graphics. For details on all graphics functions, see Using MATLAB Graphics.
Plotting Capabilities
MATLAB's basic plotting capabilities were improved and expanded in MATLAB 5.0.
Function
|
Description
|
area
|
Filled area plot.
|
bar3
|
Vertical 3-D bar chart.
|
bar3h
|
Horizontal 3-D bar chart.
|
barh
|
Horizontal bar chart.
|
pie
|
Pie chart.
|
pie3
|
Three-dimensional pie chart.
|
plotyy
|
Plot graphs with Y tick labels on left and right.
|
Filling Areas
The area
function plots a set of curves and fills the area beneath the curves.
Bar Chart Enhancements
bar3
, bar3h
, and barh
draw vertical and horizontal bar charts. These functions, together with bar
, support multiple filled bars in grouped and stacked formats.
Labels for Patches and Surfaces
legend
can label any solid-color patch and surface. You can place legends on line, bar, ribbon, and pie plots, for example.
Function
|
Description
|
box
|
Axes box.
|
datetick
|
Display dates for Axes tick labels.
|
Marker Style Enhancement
A number of new line markers are available, including, among others, a square, a diamond, and a five-pointed star. These can be specified independently from line style.
Stem Plot Enhancements
stem
and stem3
plot discrete sequence data as filled or unfilled stem plots.
Three-Dimensional Plotting Support
quiver3
displays three-dimensional velocity vectors with (u
,v
,w
) components. The ribbon
function displays data as three-dimensional strips.
Function
|
Description
|
quiver3
|
Three-dimensional quiver plot.
|
ribbon
|
Draw lines as 3-D strips.
|
stem3
|
Three-dimensional stem plot.
|
Data Visualization
MATLAB 5.0 features many new and enhanced capabilities for data visualization.
New Viewing Model
Axes camera properties control the orthographic and perspective view of the scene created by an Axes and its child objects. You can view the Axes from any location around or in the scene, as well as adjust the rotation, view angle, and target point.
New Method for Defining Patches
You can define a Patch using a matrix of faces and a matrix of vertices. Each row of the face matrix contains indices into the vertex matrix that define the connectivity of the face. Defining Patches in this way reduces memory consumption because you no longer need to specify redundant vertices.
Triangular Meshes and Surfaces
The new functions trimesh
and trisurf
create triangular meshes and surfaces from x
, y
, and z
vector data and a list of indices into the vector data.
Function
|
Description
|
trisurf
|
Triangular surface plot.
|
trimesh
|
Triangular mesh plot.
|
Improved Slicing
slice
supports an arbitrary slicing surface.
Contouring Enhancements
The contouring algorithm supports parametric surfaces and contouring on triangular meshes. In addition, clabel
rotates and inserts labels in contour plots.
Function
|
Description
|
contourf
|
Filled contour plot.
|
New zoom Options
The zoom
function supports two new options:
Graphics Presentation
MATLAB 5.0 provides improved control over the display of graphics objects.
Enhancements to Axes Objects
MATLAB 5.0 provides more advanced control for three-dimensional Axes objects. You can control the three-dimensional aspect ratio for the Axes' plot box, as well as for the data displayed in the plot box. You can also zoom in and out from a three-dimensional Axes using viewport scaling and Axes camera properties.
The axis
command supports a new option designed for viewing graphics objects in 3-D:
axis vis3d
This option prevents MATLAB from stretching the Axes to fit the size of the Figure window and otherwise altering the proportions of the objects as you change the view.
In a two-dimensional view, you can display the x-axis at the top of an Axes and the y-axis at the right side of an Axes.
Color Enhancements
colordef white
or colordef black
changes the color defaults on the root so that subsequent figures produce plots with a white or black axes background color. The figure background color is changed to be a shade of gray, and many other defaults are changed so that there will be adequate contrast for most plots. colordef none
sets the defaults to their MATLAB 4 values. In addition, a number of new colormaps are available.
Table 3-6: New Figure and Axis Color Control
Function
|
Description
|
colordef
|
Select Figure color scheme.
|
Table 3-7: New Colormaps
Function
|
Description
|
autumn
|
Shades of red and yellow colormap.
|
colorcube
|
Regularly spaced colors in RGB colorspace, plus more steps of gray, pure red, pure green, and pure blue.
|
lines
|
Colormap of colors specified by the Axes' ColorOrder property.
|
spring
|
Shades of magenta and yellow colormap.
|
summer
|
Shades of green and yellow colormap.
|
winter
|
Shades of blue and green colormap.
|
Text Object Enhancements
MATLAB 5.0 supports a subset of TeX commands. A single Text graphics object can support multiple fonts, subscripts, superscripts, and Greek symbols. See the text
function in the online MATLAB Function Reference for information about the supported TeX subset.
You can also specify multiline character strings and use normalized font units so that Text size is a fraction of an Axes' or Uicontrol's height. MATLAB supports multiline text strings using cell arrays. Simply define a string variable as a cell array with one line per cell.
Improved General Graphics Features
The MATLAB startup file sets default properties for various graphics objects so that new Figures are aesthetically pleasing and graphs are easier to understand.
Command
|
Description
|
dialog
|
Create a dialog box.
|
Z-buffering is available for fast and accurate three-dimensional rendering.
MATLAB 5.0 provides built-in menus on X Window systems. Figure MenuBar 'figure'
is supported on UNIX.
Lighting
MATLAB supports a new graphics object called a Light. You create a Light object using the light
function. Three important Light object properties are:
You cannot see Light objects themselves, but you can see their effect on any Patch and Surface objects present in the same Axes. You can control these effects by setting various Patch and Surface object properties.
AmbientStrength
, DiffuseStrength
, and SpecularStrength
control the intensity of the respective light-reflection characteristics;
SpecularColorReflectance
and SpecularExponent
provide additional control over the reflection characteristics of specular light.
The Axes AmbientLightColor
property determines the color of the ambient light, which has no direction and affects all objects uniformly. Ambient light effects occur only when there is a visible Light object in the Axes.
The Light object's Color
property determines the color of the directional light, and its Style
property determines whether the light source is a point source (Style
set to local
), which radiates from the specified position in all directions, or a light source placed at infinity (Style
set to infinite
), which shines from the direction of the specified position with parallel rays.
You can also select the algorithm used to calculate the coloring of the lit objects. The Patch and Surface EdgeLighting
and FaceLighting
properties select between no lighting, and flat, Gouraud, or Phong lighting algorithms.
print Command Revisions
The print
command has been extensively revised for MATLAB 5.0. Consult Using MATLAB Graphics for a complete description of print
command capabilities. Among the new options available for MATLAB 5.0:
Additional print Device Options
MATLAB 5.0 introduced several new device options for the print
command:
Device
|
Description
|
-dljet4
|
HP LaserJet 4 (defaults to 600 dpi)
|
-ddeskjet
|
HP DeskJet and DeskJet Plus
|
-ddjet500
|
HP Deskjet 500
|
-dcdj500
|
HP DeskJet 500C
|
-dcdj550
|
HP Deskjet 550C
|
-dpjxl
|
HP PaintJet XL color printer
|
-dpjxl300
|
HP PaintJet XL300 color printer
|
-ddnj650c
|
HP DesignJet 650C
|
-dbj200
|
Canon BubbleJet BJ200
|
-dbjc600
|
Canon Color BubbleJet BJC-600 and BJC-4000
|
-dibmpro
|
IBM 9-pin Proprinter
|
-dbmp256
|
8-bit (256-color) BMP file format
|
-dbmp16m
|
24-bit BMP file format
|
-dpcxmono
|
Monochrome PCX file format
|
-dpcx24b
|
24-bit color PCX file format, three 8-bit planes
|
-dpbm
|
Portable Bitmap (plain format)
|
-dpbmraw
|
Portable Bitmap (raw format)
|
-dpgm
|
Portable Graymap (plain format)
|
-dpgmraw
|
Portable Graymap (raw format)
|
-dppm
|
Portable Pixmap (plain format)
|
-dppmraw
|
Portable Pixmap (raw format)
|
Image Support
MATLAB 5.0 provides a number of enhancements to image support. These enhancements include:
Truecolor
In addition to indexed images, in which colors are stored as an array of indices into a colormap, MATLAB 5.0 supports truecolor images. A truecolor image does not use a colormap; instead, the color values for each pixel are stored directly as RGB (red, green, blue) triplets. In MATLAB, the CData
property of a truecolor Image object is a three-dimensional (m
-by-n
-by-3) array. This array consists of three m
-by-n
matrices (representing the red, green, and blue color planes) concatenated along the third dimension.
Reading and Writing Images
The imread
function reads image data into MATLAB arrays from graphics files in various standard formats, such as TIFF. You can then display these arrays using the image
function, which creates a Handle Graphics Image object. You can also write MATLAB image data to graphics files using the imwrite
function. imread
and imwrite
both support a variety of graphics file formats and compression schemes.
8-Bit Images
When you read an image into MATLAB using imread
, the data is stored as an array of 8-bit integers. This is a much more efficient storage method than the double-precision (64-bit) floating-point numbers that MATLAB typically uses.
The Handle Graphics Image object has been enhanced to support 8-bit CData
. This means you can display 8-bit images without having to convert the data to double precision. MATLAB 5.0 also supports a limited set of operations on these 8-bit arrays. You can view the data, reference values, and reshape the array in various ways. To perform any mathematical computations, however, you must first convert the data to double precision, using the double
function.
Note that, in order to support 8-bit images, certain changes have been made in the way MATLAB interprets image data. This table summarizes the conventions MATLAB uses:
Image Type
|
Double-Precision Data (Double Array)
|
8-Bit Data (uint8 Array)
|
Indexed (colormap)
|
Image is stored as a 2-D (m -by-n ) array of integers in the range [1,length(colormap) ]; colormap is an m-by-3 array of floating-point values in the range [0, 1].
|
Image is stored as a 2-D (m -by-n ) array of integers in the range [0, 255]; colormap is an m-by-3 array of floating-point values in the range [0, 1].
|
Truecolor (RGB)
|
Image is stored as a 3-D (m -by-n -by-3) array of floating-point values in the range [0, 1].
|
Image is stored as a 3-D (m -by-n -by-3) array of integers in the range [0, 255].
|
Note that MATLAB interprets image data very differently depending on whether it is double precision or 8-bit. The rest of this section discusses factors you should keep in mind when working with image data to avoid potential pitfalls. This information is especially important if you want to convert image data from one format to another.
Indexed Images
In an indexed image of class double
, the value 1 points to the first row in the colormap, the value 2 points to the second row, and so on. In a uint8
indexed image, there is an offset; the value 0 points to the first row in the colormap, the value 1 points to the second row, and so on. The uint8
convention is also used in graphics file formats, and enables 8-bit indexed images to support up to 256 colors. Note that when you read in an indexed image with imread
, the resulting image array is always of class uint8
. (The colormap, however, is of class double
; see below.)
If you want to convert a uint8
indexed image to double
, you need to add 1 to the result. For example:
X64 = double(X8) + 1;
To convert from double
to uint8
, you need to first subtract 1, and then use round
to ensure that all the values are integers:
X8 = uint8(round(X64 - 1));
The order of the operations must be as shown in these examples because you cannot perform mathematical operations on uint8
arrays.
When you write an indexed image using imwrite
, MATLAB automatically converts the values if necessary.
Colormaps
Colormaps in MATLAB are always m
-by-3 arrays of double-precision floating-point numbers in the range [0, 1]. In most graphics file formats, colormaps are stored as integers, but MATLAB does not support colormaps with integer values. imread
and imwrite
automatically convert colormap values when reading and writing files.
Truecolor Images
In a truecolor image of class double
, the data values are floating-point numbers in the range [0, 1]. In a truecolor image of class uint8
, the data values are integers in the range [0, 255].
If you want to convert a truecolor image from one data type to the other, you must rescale the data. For example, this call converts a uint8
truecolor image to double
:
RGB64 = double(RGB8)/255;
This call converts a double
truecolor image to uint8
:
RGB8 = uint8(round(RGB*255));
The order of the operations must be as shown in these examples, because you cannot perform mathematical operations on uint8
arrays.
When you write a truecolor image using imwrite
, MATLAB automatically converts the values if necessary.
New and Enhanced Handle Graphics Object Properties
This section lists new graphics object properties supported in MATLAB 5.0. It also lists graphics properties whose behavior has changed significantly.
Using MATLAB Graphics provides a more detailed description of each property.
Table 3-8: Properties of All Graphics Objects
Property
|
Description
|
BusyAction
|
Controls events that potentially interrupt executing callback routines.
|
Children
|
Enhanced behavior allows reordering of child objects.
|
CreateFcn
|
A callback routine that executes when MATLAB creates a new instance of the specific type of graphics object.
|
DeleteFcn
|
A callback routine that executes when MATLAB deletes the graphics object.
|
HandleVisibility
|
Controls scope of handle visibility.
|
Interruptible
|
On by default.
|
Parent
|
Enhanced behavior allows reparenting of graphics objects.
|
Selected
|
Indicates whether graphics object is in selected state.
|
SelectionHighlight
|
Determines if graphics objects provide visual indication of selected state.
|
Tag
|
User-specified object label.
|
Table 3-9: Axes Properties
Property
|
Description
|
AmbientLightColor
|
Color of the surrounding light illuminating all Axes child objects when a Light object is present.
|
CameraPosition
|
Location of the point from which the Axes is viewed.
|
CameraPositionMode
|
Automatic or manual camera positioning.
|
CameraTarget
|
Point in Axes viewed from camera position.
|
CameraTargetMode
|
Automatic or manual camera target selection.
|
CameraUpVector
|
Determines camera rotation around the viewing axis.
|
CameraUpVectorMode
|
Default or user-specified camera orientation.
|
CameraViewAngle
|
Angle determining the camera field of view.
|
CameraViewAngleMode
|
Automatic or manual camera field of view selection.
|
DataAspectRatio
|
Relative scaling of x-, y-, and z-axis data units.
|
DataAspectRatioMode
|
Automatic or manual axis data scaling.
|
FontUnits
|
Units used to interpret the FontSize property (allowing normalized text size).
|
Layer
|
Draw axis lines below or above child objects.
|
NextPlot
|
Enhanced behavior supports add , replace , and replacechildren options.
|
PlotBoxAspectRatio
|
Relative scaling of Axes plot box.
|
PlotBoxAspectRatioMode
|
Automatic or manual selection of plot box scaling.
|
Projection
|
Select orthographic or perspective projection type.
|
TickDirMode
|
Automatic or manual selection of tick mark direction (allowing you to change view and preserve the specified TickDir ).
|
XAxisLocation
|
Locate x-axis at bottom or top of plot.
|
YAxisLocation
|
Locate y-axis at left or right side of plot.
|
Table 3-10: Figure Properties
Property
|
Description
|
CloseRequestFcn
|
Callback routine executed when you issue a close command on a Figure.
|
Dithermap
|
Colormap used for truecolor data on pseudocolor displays.
|
DithermapMode
|
Automatic dithermap generation.
|
IntegerHandle
|
Integer or floating-point Figure handle.
|
NextPlot
|
Enhanced behavior supports add , replace , and replacechildren options.
|
PaperPositionMode
|
WYSIWYG printing of Figure.
|
PointerShapeCData
|
User-defined pointer data.
|
PointerShapeHotSpot
|
Active point in custom pointer.
|
Renderer
|
Select painters or Z-buffer rendering.
|
RendererMode
|
Enable MATLAB to select best renderer automatically.
|
Resize
|
Determines if Figure window is resizeable.
|
ResizeFcn
|
Callback routine executed when you resize the Figure window.
|
Table 3-11: Image Properties
Property
|
Description
|
CData
|
Enhanced behavior allows truecolor (RGB values) specification.
|
CDataMapping
|
Select direct or scaled interpretation of indexed colors.
|
EraseMode
|
Control drawing and erasing of Image objects.
|
Table 3-12: Light Properties
Property
|
Description
|
Color
|
Color of the light source.
|
Position
|
Place the light source within Axes space.
|
Style
|
Select infinite or local light source.
|
Table 3-13: Line Properties
Property
|
Description
|
Mark er
|
The marker symbol to use at data points (markers are separate from line style).
|
MarkerEdgeColor
|
The color of the edge of the marker symbol.
|
MarkerFaceColor
|
The color of the face of filled markers.
|
Table 3-14: Patch Properties
Property
|
Description
|
AmbientStrength
|
The strength of the Axes ambient light on the particular Patch object.
|
CData
|
Enhanced behavior allows truecolor (RGB values) specification.
|
CDataMapping
|
Select direct or scaled interpretation of indexed colors.
|
DiffuseStrength
|
Strength of the reflection of diffuse light from Light objects.
|
FaceLightingAlgorithm
|
Lighting algorithm used for Patch faces.
|
Faces
|
The vertices connected to define each face.
|
FaceVertexCData
|
Color specification when using the Faces and Vertices properties to define a Patch.
|
LineStyle
|
Type of line used for edges.
|
Marker
|
Symbol used at vertices.
|
MarkerEdgeColor
|
The color of the edge of the marker symbol.
|
MarkerFaceColor
|
The color of the face of filled markers.
|
MarkerSize
|
Size of the marker.
|
NormalMode
|
MATLAB generated or user-specified normal vectors.
|
SpecularColorReflectance
|
Control the color of the specularly reflected light from Light objects.
|
SpecularExponent
|
Control the shininess of the Patch object.
|
SpecularStrength
|
Strength of the reflection of specular light from Light objects.
|
VertexNormals
|
Definition of the Patch's normal vectors.
|
Vertices
|
The coordinates of the vertices defining the Patch.
|
Table 3-16: Surface Properties
Property
|
Description
|
AmbientStrength
|
The strength of the Axes ambient light on the particular Surface object.
|
CData
|
Enhanced behavior allows truecolor (RGB values) specification.
|
CDataMapping
|
Selects direct or scaled interpretation of indexed colors.
|
DiffuseStrength
|
Strength of the reflection of diffuse light from Light objects.
|
FaceLightingAlgorithm
|
Lighting algorithm used for Surface faces.
|
Marker
|
Symbol used at vertices.
|
MarkerEdgeColor
|
The color of the edge of the marker symbol.
|
MarkerFaceColor
|
The color of the face of filled markers.
|
MarkerSize
|
Size of the marker.
|
NormalMode
|
MATLAB generated or user-specified normal vectors.
|
SpecularColorReflectance
|
Control the color of the specularly reflected light from Light objects.
|
SpecularExponent
|
Control the shininess of the Surface object.
|
SpecularStrength
|
Strength of the reflection of specular light from Light objects.
|
VertexNormals
|
Definition of the Surface's normal vectors.
|
Vertices
|
The coordinates of the vertices defining the Surface.
|
Table 3-17: Text Properties
Property
|
Description
|
FontUnits
|
Select the units used to interpret the
FontSize property (allowing normalized text size).
|
Interpreter
|
Allows MATLAB to interpret certain characters as TeX commands.
|
Table 3-18: Uicontrol Properties
Property
|
Description
|
Enable
|
Enable or disable (gray out) uicontrols.
|
FontAngle
|
Select character slant.
|
FontName
|
Select font family.
|
FontSize
|
Select font size.
|
FontUnits
|
Select the units used to interpret the
FontSize property (allowing normalized text size).
|
FontWeight
|
Select the weight of text characters.
|
ListboxTop
|
Select the listbox item to display at the top of the listbox.
|
SliderStep
|
Select the size of the slider step.
|
Style
|
Enhanced to include listbox device.
|
Table 3-19: Uimenu Properties
Property
|
Description
|
Enable
|
Enable or disable (gray out) uicontrols.
|
Improvements to Graphical User Interfaces (GUIs)
General GUI Enhancements
MATLAB 5.0 provides general enhancements that are useful in the GUI area:
MATLAB 5.0 provides features that make it easier to create MATLAB GUIs. Major enhancements include List Box objects to display and select one or more list items. You can also create modal or non-modal error, help, and warning message boxes. In addition, uicontrol edit boxes support multiline text.
MATLAB 5.0 also provides more flexibility in callback routines. You can specify callbacks that execute after creating, changing, and deleting an object.
Function
|
Description
|
uiresume
|
Resume suspended M-file execution.
|
uiwait
|
Block program execution.
|
waitfor
|
Block execution until a condition is satisfied.
|
Guide
Guide is a GUI design tool. The individual pieces of the Guide environment are designed to work together, but they can also be used individually. For example, there is a Property Editor (invoked by the command propedit
) that allows you to modify any property of any Handle Graphics object, from a figure to a line. Point the Property Editor at a line and you can change its color, position, thickness, or any other line property.
The Control Panel is the centerpiece of the Guide suite of tools. It lets you "control" a figure so that it can be easily modified by clicking and dragging. As an example, you might want to move a button from one part of a figure to another. From the Control Panel you put the button's figure into an editable state, and then it's simply a matter of dragging the button into the new position. Once a figure is editable, you can also add new uicontrols, uimenus, and plotting axes.
Tool
|
Command
|
Description
|
Control Panel
|
guide
|
Control figure editing.
|
Property Editor
|
propedit
|
Modify object properties.
|
Callback Editor
|
cbedit
|
Modify object callbacks.
|
Alignment Tool
|
align
|
Align objects.
|
Menu Editor
|
menuedit
|
Modify figure menus.
|
Enhanced Application Program Interface (API)
The MATLAB 5.0 API introduces data types and functions not present in MATLAB 4. This section summarizes the important changes in the API. For details on any of these topics, see the MATLAB Application Program Interface Guide.
New Fundamental Data Type
The MATLAB 4 Matrix
data type is obsolete. MATLAB 5.0 programs use the mxArray
data type in place of Matrix
. The mxArray
data type has extra fields to handle the richer data constructs of MATLAB 5.0.
Functions that expected Matrix
arguments in MATLAB 4 expect mxArray
arguments in MATLAB 5.0.
New Functions
The MATLAB 5.0 API introduced many new functions that work with the C language to support MATLAB 5.0 features.
Support for Structures and Cells
MATLAB 5.0 introduced structure arrays and cell arrays. Therefore, the MATLAB 5.0 API introduced a broad range of functions to create structures and cells, as well as functions to populate and analyze them. See Chapter 4 of this document for a complete listing of these functions.
Support for Multidimensional Arrays
The MATLAB 4 Matrix
data type assumed that all matrices were two-dimensional. The MATLAB 5.0 mxArray
data type supports arrays of two or more dimensions. The MATLAB 5.0 API provides two different mxCreate
functions that create either a two-dimensional or a multidimensional mxArray
.
In addition, MATLAB 5.0 introduces several functions to get and set the number and length of each dimension in a multidimensional mxArray
.
Support for Nondouble Precision Data
The MATLAB 4 Matrix
data type represented all numerical data as double-precision floating-point numbers. The MATLAB 5.0 mxArray
data type can store numerical data in six different integer formats and two different floating-point formats.
Note: Although the MATLAB API supports these different data representations, MATLAB itself does not currently provide any operations or functions that work with nondouble-precision data. Nondouble precision-data may be viewed, however.
Enhanced Debugging Support
MATLAB 5.0 includes more powerful tools for debugging C MEX-files. The
-argcheck
option to the mex
script provides protection against accidental misuse of API functions (such as passing NULL
pointers). In addition, there is increased documentation on troubleshooting common problems.
Enhanced Compile Mechanism
MATLAB 5.0 replaced the old cmex
and fmex
scripts with mex
, which compile C or Fortran MEX-files. All compiler-specific information was moved to easily readable and highly configurable options files. The mex
script has a configurable set of flags across platforms and can be accessed from within MATLAB via the mex.m
M-file.
MATLAB 4 Feature Unsupported in MATLAB 5.0
Non-ANSI C Compilers
MATLAB 4 let you compile MATLAB applications with non-ANSI C compilers. MATLAB 5.0 requires an ANSI C compiler.
New Platform-Specific Features
Microsoft Windows
Path Browser
The Path Browser lets you view and modify the MATLAB search path. All changes take effect in MATLAB immediately.
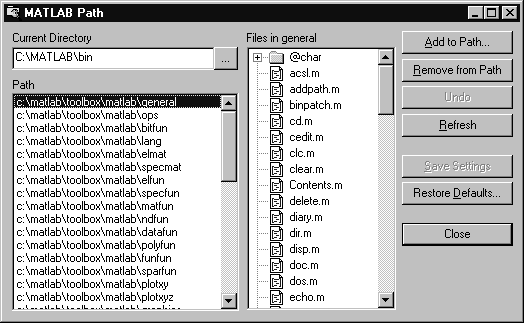
Workspace Browser
The Workspace Browser lets you view the contents of the current MATLAB workspace. It provides a graphical representation of the traditional whos
output. In addition, you can clear workspace variables and rename them.
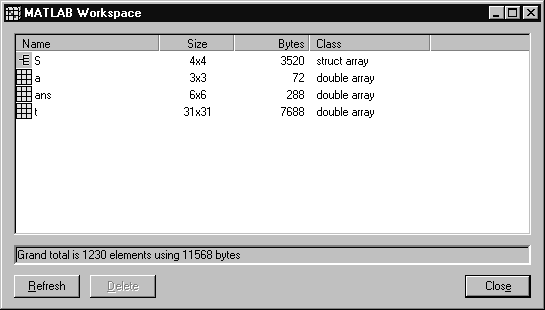
M-File Editor/Debugger
The graphical M-file Editor/Debugger allows you to set breakpoints and single-step through M-code. The M-file Editor/Debugger starts automatically when a breakpoint is hit. When MATLAB is installed, this program becomes the default editor.
Command Window Toolbar
A toolbar is optionally present for the Command Window. The toolbar provides single-click access to several commonly used operations:

New Dialog Boxes
New Preferences dialog boxes are accessible through the File menu. Some of these were previously available through the Options menu in MATLAB 4. There are three categories of preferences:
16-bit Stereo Sound
MATLAB 5.0 supports 16-bit stereo sound on the Windows platform.
Macintosh
Two new features available on the Macintosh platform are:
User Interface Enhancements
- Optional toolbars in the Command Window, Editor windows, and M-file debugger allow rapid access to commonly used features.

- Color syntax highlighting in the Command Window, Editor windows, and M-file debugger provides visual cues for identifying blocks of code, comments, and strings.
- Almost all lists and text items in the Command Window, Editor, Path Browser, Workspace Browser, M-file debugger, and Command History Window have optional dynamic or "live" scrolling; the display is scrolled as the scroll box of a scrollbar is moved.
- Macintosh Drag and Drop is supported throughout MATLAB for rapid and easy exchange of text between windows.
Command Window Features
Command History Window
The Command History window contains a list of all commands executed from the Command Window. Commands are saved between MATLAB sessions, so you can select and execute a group of commands from a previous day's work to continue quickly from where you left off.
Path Browser
The Path Browser provides an intuitive, easy-to-use graphical interface for viewing and modifying the MATLAB search path. You can reorder or modify the search path simply by dragging items in the path list. Similarly, you can change the current MATLAB directory by dragging any folder into the current MATLAB directory area
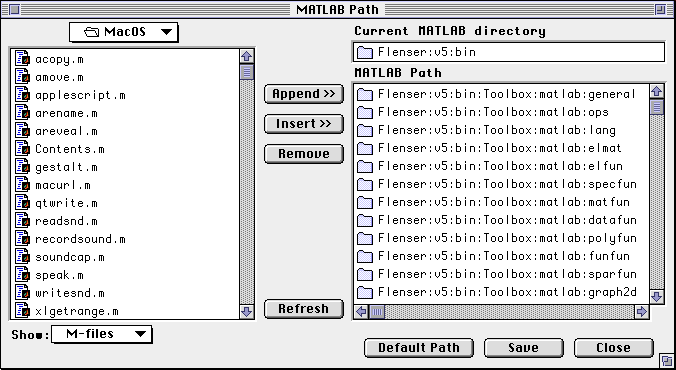
.
Workspace Browser
The Workspace Browser allows you to view the contents of the current MATLAB workspace. It provides a graphic representation of the traditional whos
output. You can delete variables from the workspace and sort the workspace by various criteria. Double-clicking on a workspace variable displays that variable's contents in the Command Window.
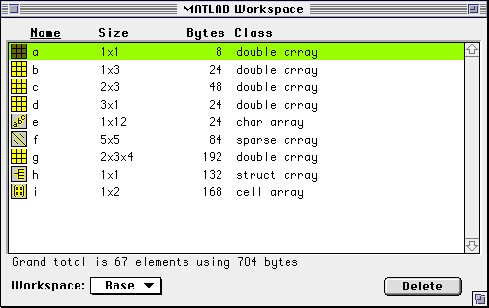
M-File Debugger
MATLAB 5.0 includes a graphical M-file debugger, which allows you to set breakpoints and single-step through M-code. Selecting text in the debugger window and pressing the Enter (not the Return) key evaluates that text in the Command Window.
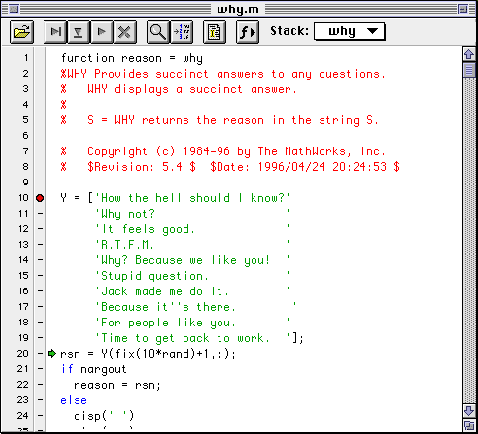
Editor Features
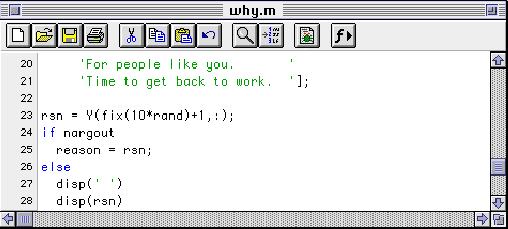
UNIX Workstations
Figure Window Toolbar
The MATLAB 5.0 Figure window provides a toolbar with a File pull-down menu. Selecting the Print option on the File menu activates a set of push buttons that allows easy setting of the most frequently used print options.
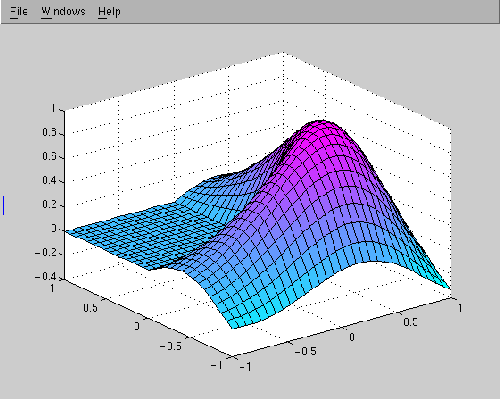
Path Editor
The editpath
command displays a GUI that allows you to view and modify your MATLAB search path.
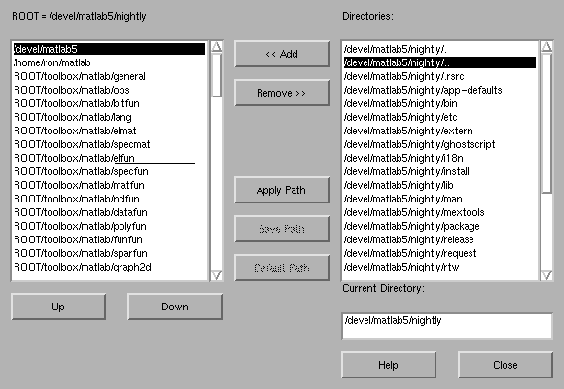
Simplified Installation Procedure
The MATLAB 5.0 installation procedure uses a GUI to select or deselect products and platforms.
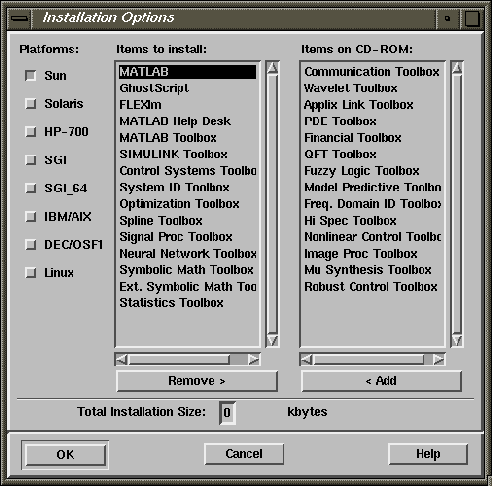
[ Previous | Help Desk | Next ]